Ah, XML! An integral part of the digital world we so love. While the majority of developers are shifting towards JSON, XML still has its significant use cases, especially in configurations, web services, and document storage. If you’re an iOS developer, understanding XML parsing is indispensable. Let’s decode this essential skill in iOS programming.
The Basics of XML and Its Importance in iOS Development
Every techie will probably tell you that XML (eXtensible Markup Language) is a markup language designed to store and transport data. But why should an iOS developer bother?
- Configurations and Settings: Many apps use XML to store configuration data. Easier to read and modify than plists!;
- Data Interchange: Exchanging data between an iOS device and a server? Sometimes, XML is the answer!;
- Compatibility: Some legacy systems still spit out XML, so understanding its workings keeps you versatile.
But enough of the whys; let’s get to the hows!
Parsing XML in iOS: Getting Started
To be honest, XML parsing in iOS isn’t rocket science. With Apple’s Foundation framework, the tools are right at your fingertips.
Using ‘XMLParser’
Did you know Apple provides a built-in class to parse XML? Say hello to XMLParser.
Steps to use ‘XMLParser’:
- Initialization: Start by initializing the ‘XMLParser’ object with your XML data;
- Delegate Assignment: Assign a delegate to handle the parsing events;
- Starting the Parsing: Once you’ve set the delegate, call the ‘parse’ method.
Sounds too good? Here’s a tiny catch. ‘XMLParser’ uses an event-driven approach. It means as it reads through the XML, it triggers events like “found an element” or “found characters”. Your job? Handle these events!
DOM vs SAX Parsing
At this juncture, you might be wondering about two popular XML parsing techniques: DOM (Document Object Model) and SAX (Simple API for XML). Which one’s for you?
DOM Parsing:
- Loads entire XML into memory;
- Easy to navigate nodes;
- Consumes more memory (a potential downside for huge XMLs).
SAX Parsing:
- Reads XML sequentially;
- Uses callbacks for nodes;
- Memory efficient, but not easy to navigate.
For iOS, ‘XMLParser’ uses the SAX approach. If DOM is what you seek, consider third-party libraries.
Working with Third-Party Libraries
Sometimes, Apple’s tools might not fit the bill. Maybe you want extra features, or maybe you’re looking for a different parsing approach. Enter third-party libraries!
SwiftXMLParser
A popular choice among iOS developers. Why? Because it’s Swift-friendly, lightweight, and super-fast. Plus, it supports both DOM and SAX!
Alamofire & AEXML
Ever heard of Alamofire? The popular networking library for iOS? Pair it with AEXML, and you’ve got a killer combination for fetching and parsing XML!
XML Parsing Pitfalls and How to Avoid Them
XML parsing isn’t without its quirks. Here are some challenges you might face:
- Large XML Documents: Can be memory intensive. Always ensure you’re choosing the right parsing technique;
- Incorrect XML Format: A missing tag? Parsing fails! Always validate your XML;
- Thread Management: XML parsing can be time-consuming. Always perform it in a background thread to ensure UI responsiveness.
Best Practices in XML Parsing
- Always Use Error Handling: Implement robust error checks;
- Test with Different XML Structures: To ensure flexibility;
- Mind the Memory: Especially with large XML documents.
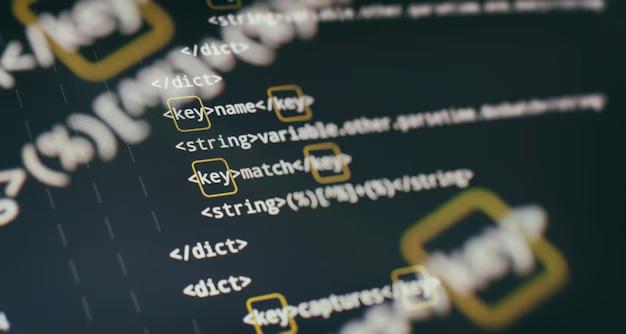
Tinting the Status Bar in iOS with XML Integration
The status bar, that small space at your device’s top, can be visually customized to enhance an app’s aesthetics. When paired with XML-driven themes, its visual appeal can be dynamic and striking.
Why Tint the Status Bar?
- Consistency: Align the status bar with XML-driven themes;
- Branding: Infuse brand colors subtly;
- User Experience: Elevate aesthetics for user satisfaction.
Conclusion
XML parsing might seem daunting at first, but with the right tools and knowledge, it’s a breeze. Whether you’re using Apple’s built-in tools or diving into third-party libraries, mastering XML parsing can significantly boost your iOS development skills.
FAQs
It depends on the use-case. JSON is lightweight and easy to parse, but XML has its merits, especially in configurations and legacy systems.
Absolutely! Apple’s XMLParser is sufficient for most needs.
Popular choices include SwiftXMLParser and AEXML, depending on your requirements.
Parsing might be consuming too much memory or blocking the main thread. Consider using background threads or changing your parsing technique.
Several online tools can validate XML. Additionally, some third-party libraries provide validation functionalities.